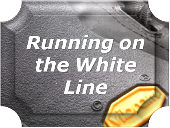
Take this button:
Take the code box (shown above) for this button:
<div align=”center”>
<form> <textarea rows=”6″ cols=”19″ readonly=”readonly”><a href="http://www.runningonthewhiteline.com/" target="_blank"><img alt="Running on the White Line" src="http://i988.photobucket.com/albums/af5/nixiao/WhiteLine-2.png" border="0" /></a></textarea></form>
</div>
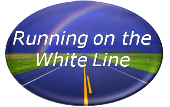
Take this button:
Take the code box (shown above) for this button:
<div align=”center”>
<form> <textarea rows=”6″ cols=”19″ readonly=”readonly”><a href="http://www.runningonthewhiteline.com/" target="_blank"><img alt="Running on the White Line" src="http://i988.photobucket.com/albums/af5/nixiao/whiteline3-1.png" border="0" /></a></textarea></form>
</div>
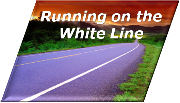
Take this button:
Take the code box (shown above) for this button:
<div align=”center”>
<form> <textarea rows=”6″ cols=”19″ readonly=”readonly”><a href="http://www.runningonthewhiteline.com/" target="_blank"><img alt="Running on the White Line" src="http://i988.photobucket.com/albums/af5/nixiao/whiteline2-1.png" border="0" /></a></textarea></form>
</div>
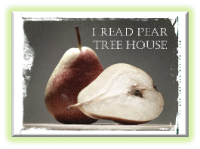
Take this button:
Take the code box (shown above) for this button:
<div align=”center”>
<form> <textarea rows=”6″ cols=”19″ readonly=”readonly”><a href="http://notesfromthepeartree.blogspot.com/" target="_blank"><img alt="Notes from the Pear Tree House" src="http://i988.photobucket.com/albums/af5/nixiao/peartree2-1-2.png" border="0" /></a></textarea></form>
</div>
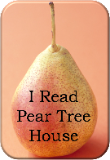
Take this button:
Take the code box (shown above) for this button:
<div align=”center”>
<form> <textarea rows=”6″ cols=”19″ readonly=”readonly”><a href="http://notesfromthepeartree.blogspot.com/" target="_blank"><img alt="Notes from the Pear Tree House" src="http://i988.photobucket.com/albums/af5/nixiao/peartree3-1-1.png" border="0" /></a></textarea></form>
</div>
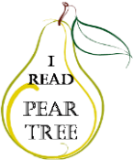
Take this button:
Take the code box (shown above) for this button:
<div align=”center”>
<form> <textarea rows=”6″ cols=”19″ readonly=”readonly”><a href="http://notesfromthepeartree.blogspot.com/" target="_blank"><img alt="Notes from the Pear Tree House" src="http://i988.photobucket.com/albums/af5/nixiao/peartree1-1-1-1.png" border="0" /></a></textarea></form>
</div>
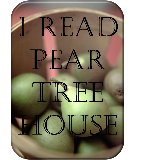
Take this button:
Take the code box (shown above) for this button:
<div align=”center”>
<form> <textarea rows=”6″ cols=”19″ readonly=”readonly”><a href="http://notesfromthepeartree.blogspot.com/" target="_blank"><img alt="Notes from the Pear Tree House" src="http://i988.photobucket.com/albums/af5/nixiao/peartree4-1-2.png" border="0" /></a></textarea></form>
</div>
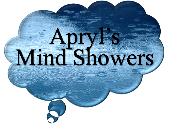
Take this button:
Take the code box (shown above) for this button:
<div align=”center”>
<form> <textarea rows=”6″ cols=”19″ readonly=”readonly”><a href="http://aprylsmindshowers.blogspot.com/" target="_blank"><img alt="Apryl's Mind Showers" src="http://i988.photobucket.com/albums/af5/nixiao/apryl3-1.png" border="0" /></a></textarea></form>
</div>
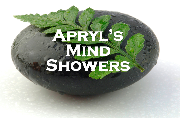
Take this button:
Take the code box (shown above) for this button:
<div align=”center”>
<form> <textarea rows=”6″ cols=”19″ readonly=”readonly”><a href="http://aprylsmindshowers.blogspot.com/" target="_blank"><img alt="Apryl's Mind Showers" src="http://i988.photobucket.com/albums/af5/nixiao/apryl3-1.png" border="0" /></a></textarea></form>
</div>
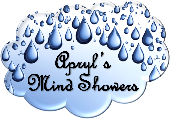
Take this button:
Take the code box (shown above) for this button:
<div align=”center”>
<form> <textarea rows=”6″ cols=”19″ readonly=”readonly”><a href="http://aprylsmindshowers.blogspot.com/" target="_blank"><img alt="Apryl's Mind Showers" src="http://i988.photobucket.com/albums/af5/nixiao/Apryl-1.png" border="0" /></a></textarea></form>
</div>